Java8新特性之函数式接口
# 什么是函数式接口
如果一个接口中,只声明了一个抽象方法,则此接口就称为函数式接口。可以通过增加@FunctionalInterface注解进行编译时判断。
# JDK提供的函数式接口
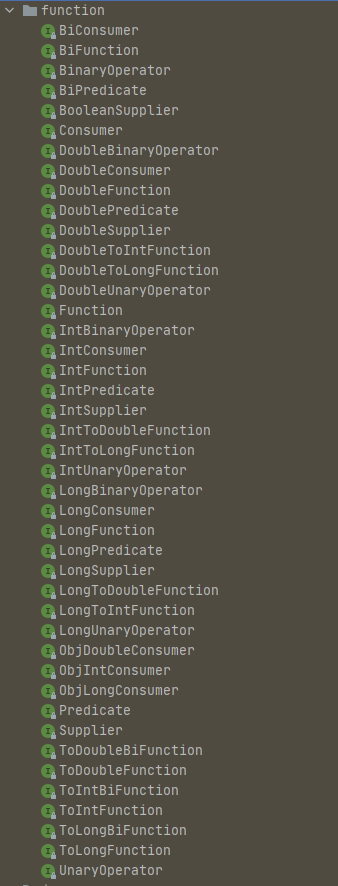
可以看到JDK提供了大量的函数式接口
# Consumer
故名思意就是提供给你一个对象然后你只需要做对应的处理,也可以说是消费即可。
@FunctionalInterface
public interface Consumer<T> {
void accept(T t);
default Consumer<T> andThen(Consumer<? super T> after) {
Objects.requireNonNull(after);
return (T t) -> { accept(t); after.accept(t); };
}
}
1
2
3
4
5
6
7
8
9
10
2
3
4
5
6
7
8
9
10
# Supplier
可以当作一个供应商一样,只负责传值
@FunctionalInterface
public interface Supplier<T> {
T get();
}
1
2
3
4
2
3
4
# Predicate
断言,也就是传入一个值返回对应的true/false,也可以链式调用
@FunctionalInterface
public interface Predicate<T, R> {
boolean test(T t);
}
public class FunctionTest {
public static void main(String[] args) {
System.out.println(aAndB(n -> n < 101, n -> n > 10));
}
public static boolean aAndB(Predicate<Integer> a, Predicate<Integer> b){
return a.and(b).test(100);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
2
3
4
5
6
7
8
9
10
11
12
13
14
15
# Function
也就是通过接受一个值,处理过后返回一个值
@FunctionalInterface
public interface Function<T, R> {
R apply(T t);
}
1
2
3
4
2
3
4
# 自定义函数式接口
在JDK中,只要接口只有一个抽象方法即可当作函数式接口,也就是说我们可以不加@FunctionalInterface
public interface MyConsumer {
void doIt();
}
1
2
3
2
3
当然如果加上了则会在编译的时候自动判断是否定义正确的。
在 GitHub 编辑此页 (opens new window)
上次更新: 2024/02/25, 12:11:11